Snake Game In Python

Snake Growth Implementation
Physics of Snake Growth
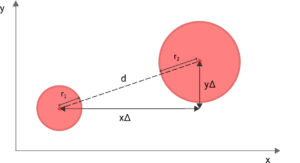
Algorithm Collision
Algorithm: HandleCollision(snake, food):
Inputs:
- snake: The snake object containing segments
- food: The position of the food
1. Get the position of the snake's head (head_position).
2. If head_position is equal to food:
- There is a collision.
- Add a new segment to the snake's body at the head position.
- Generate a new position for the food.
- Update the score or any relevant game state.
Example Implementation in Python:
def handle_collision(snake, food):
# Get the position of the snake's head
head_position = snake[0]
# Check for collision
if head_position == food:
# Add a new segment to the snake's body at the head position
snake.insert(0, head_position)
# Generate a new position for the food (random or predetermined logic)
food = generate_new_food_position()
# Update the score or any relevant game state
update_score()
return snake, food
Initializing the Game
import pygame # Import the pygame module
import random # Import the random module
# Initialize Pygame
pygame.init() # Initialize Pygame
# Set up the screen
screen_width = 800 # Set the width of the screen
screen_height = 600 # Set the height of the screen
screen = pygame.display.set_mode((screen_width, screen_height)) # Create the game window
pygame.display.set_caption("Snake Game") # Set the title of the game window
Define colors and constants:
# Colors
WHITE = (255, 255, 255) # Define the color white
BLACK = (0, 0, 0) # Define the color black
RED = (255, 0, 0) # Define the color red
GREEN = (0, 255, 0) # Define the color green
# Snake constants
BLOCK_SIZE = 20 # Set the size of the snake blocks
SNAKE_SPEED = 10 # Set the speed of the snake
Define Snake And Create Food
# Define snake
snake = [(screen_width / 2, screen_height / 2)] # Initialize the snake with its starting position at the center of the screen
snake_direction = (1, 0) # Initial direction: right
# Create food
food = (random.randrange(1, (screen_width // BLOCK_SIZE)) * BLOCK_SIZE, # Generate random x-coordinate for food
random.randrange(1, (screen_height // BLOCK_SIZE)) * BLOCK_SIZE) # Generate random y-coordinate for food
Define Ball class
We define a class Ball
to represent the game ball. The class includes methods for drawing the ball, moving it, and checking for collisions with the paddles and screen boundaries.
# Define ball
class Ball: # Define a class for the ball
def __init__(self): # Initialize the ball object
self.rect = pygame.Rect(screen_width // 2 - BALL_SIZE // 2, screen_height // 2 - BALL_SIZE // 2, BALL_SIZE, BALL_SIZE) # Create a rectangular ball
self.direction_x = random.choice([-1, 1]) # Set the initial horizontal direction of the ball
self.direction_y = random.choice([-1, 1]) # Set the initial vertical direction of the ball
def draw(self): # Method to draw the ball
pygame.draw.rect(screen, WHITE, self.rect) # Draw the ball on the screen
def move(self): # Method to move the ball
self.rect.x += self.direction_x * BALL_SPEED_X # Move the ball horizontally
self.rect.y += self.direction_y * BALL_SPEED_Y # Move the ball vertically
def check_collision(self): # Method to check for collisions
if self.rect.colliderect(player_paddle.rect) or self.rect.colliderect(ai_paddle.rect): # Check collision with paddles
self.direction_x *= -1 # Reverse the horizontal direction of the ball
if self.rect.top <= 0 or self.rect.bottom >= screen_height: # Check collision with top or bottom of the screen
self.direction_y *= -1 # Reverse the vertical direction of the ball
Main Game Loop
# Main game loop
running = True # Flag to control the main game loop
while running: # Main game loop
# Event handling
for event in pygame.event.get(): # Loop through all events
if event.type == pygame.QUIT: # Check if the user clicked the close button
running = False # Set the running flag to False to exit the loop
elif event.type == pygame.KEYDOWN: # Check if a key is pressed
if event.key == pygame.K_UP and snake_direction[1] == 0: # Check if the UP arrow key is pressed and the snake is not moving downwards
snake_direction = (0, -1) # Change snake's direction to up
elif event.key == pygame.K_DOWN and snake_direction[1] == 0: # Check if the DOWN arrow key is pressed and the snake is not moving upwards
snake_direction = (0, 1) # Change snake's direction to down
elif event.key == pygame.K_LEFT and snake_direction[0] == 0: # Check if the LEFT arrow key is pressed and the snake is not moving rightwards
snake_direction = (-1, 0) # Change snake's direction to left
elif event.key == pygame.K_RIGHT and snake_direction[0] == 0: # Check if the RIGHT arrow key is pressed and the snake is not moving leftwards
snake_direction = (1, 0) # Change snake's direction to right
Move Snake
# Move snake
if not game_over: # Check if the game is not over
x, y = snake[0] # Get the position of the snake's head
x += snake_direction[0] * BLOCK_SIZE # Move snake's head horizontally
y += snake_direction[1] * BLOCK_SIZE # Move snake's head vertically
# Check if snake collided with borders
if x < 0 or x >= screen_width or y < 0 or y >= screen_height: # Check if the snake hits the screen borders
game_over = True # Set game over flag to True
# Check if snake collided with itself
if (x, y) in snake[1:]: # Check if the snake's head hits its body
game_over = True # Set game over flag to True
# Check if snake ate food
if (x, y) == food: # Check if the snake's head reaches the food
food = (random.randrange(1, (screen_width // BLOCK_SIZE)) * BLOCK_SIZE, # Generate new random x-coordinate for food
random.randrange(1, (screen_height // BLOCK_SIZE)) * BLOCK_SIZE) # Generate new random y-coordinate for food
score += 1 # Increment the score
else:
snake.pop() # Remove the tail block of the snake
snake.insert(0, (x, y)) # Insert the new head position into the snake
Draw Everything
# Draw everything
screen.fill(BLACK) # Fill the screen with black color
for segment in snake: # Loop through each segment of the snake
pygame.draw.rect(screen, GREEN, (segment[0], segment[1], BLOCK_SIZE, BLOCK_SIZE)) # Draw the snake
pygame.draw.rect(screen, RED, (food[0], food[1], BLOCK_SIZE, BLOCK_SIZE)) # Draw the food
# Display score
font = pygame.font.SysFont(None, 24) # Create a font object
score_text = font.render("Score: " + str(score), True, WHITE) # Render the score text
screen.blit(score_text, (10, 10)) # Display the score on the screen
# Display game over message
if game_over: # Check if the game is over
game_over_text = font.render("Game Over! Press SPACE to restart.", True, WHITE) # Render the game over text
screen.blit(game_over_text, (screen_width // 2 - game_over_text.get_width() // 2,
screen_height // 2 - game_over_text.get_height() // 2)) # Display the game over text
Update, Control And Restart The Game
# Update the display
pygame.display.flip() # Update the display
# Control the frame rate
clock.tick(SNAKE_SPEED) # Limit the frame rate
# Restart game if game over and SPACE key is pressed
keys = pygame.key.get_pressed() # Get all pressed keys
if game_over and keys[pygame.K_SPACE]: # Check if the game is over and the SPACE key is pressed
snake = [(screen_width / 2, screen_height / 2)] # Reset the snake's position
snake_direction = (1, 0) # Reset the snake's direction to right
food = (random.randrange(1, (screen_width // BLOCK_SIZE)) * BLOCK_SIZE, # Generate new random x-coordinate for food
random.randrange(1, (screen_height // BLOCK_SIZE)) * BLOCK_SIZE) # Generate new random y-coordinate for food
score = 0 # Reset the score to zero
game_over = False # Reset the game over flag
# Quit Pygame
pygame.quit() # Quit Pygame
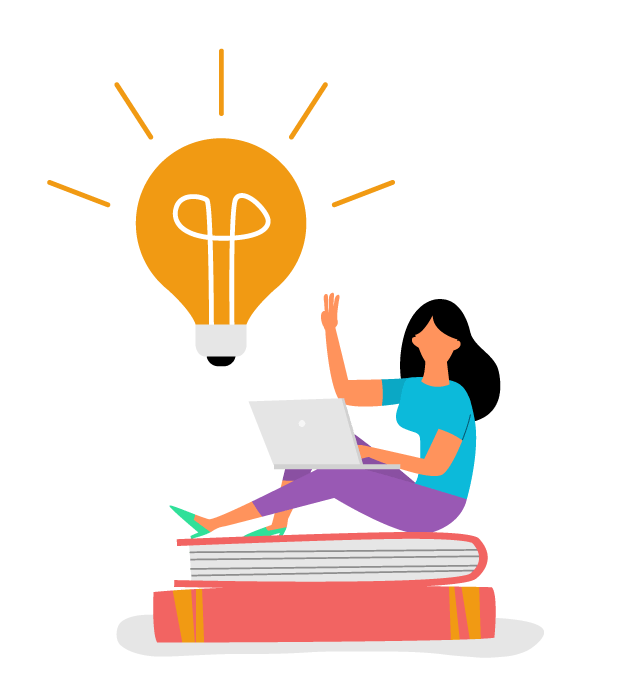
Conclusion
Post Views: 112
Tag
python
2 comments