Livre: Construis ton propre Hoverboard

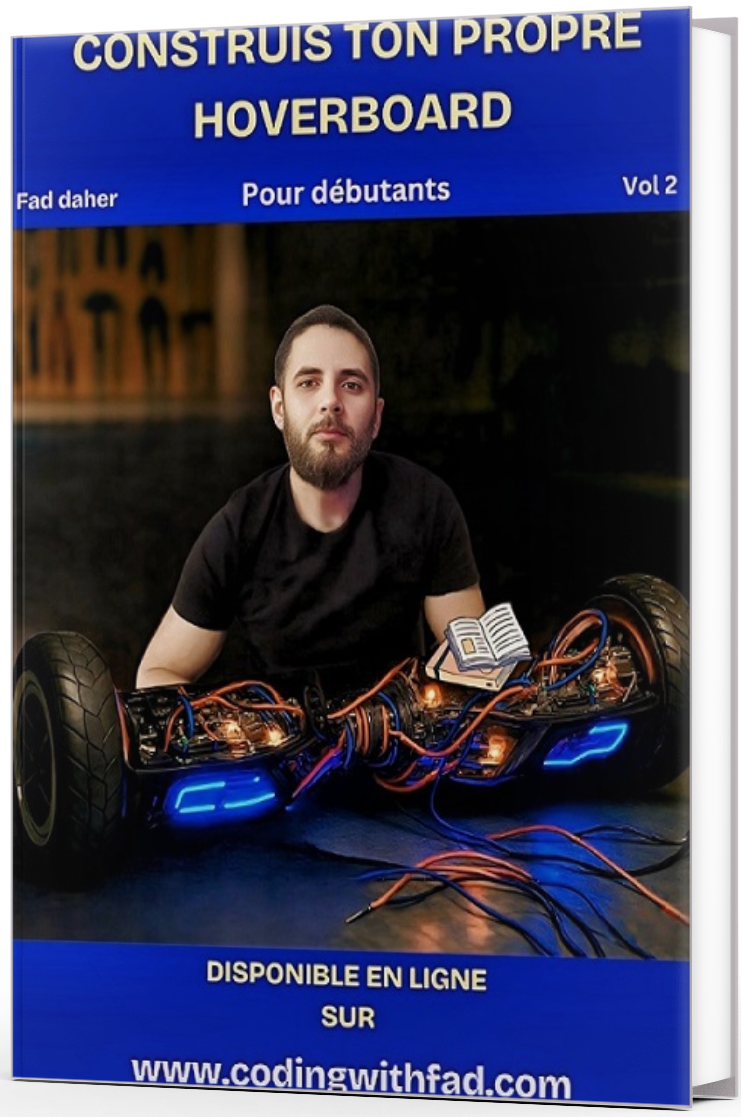
19.90€
Environ 13 053.55 XOF F CFA
Obtenir maintenantEn passant commande, vous obtenez une licence d’accès à ce contenu. Toute reproduction, distribution ou copie sans autorisation est strictement interdite.
📖 Découvrez comment construire votre propre Hoverboard de manière simple et amusante !
Ce guide est conçu pour ceux qui souhaitent créer leur propre hoverboard, qu’ils soient débutants ou passionnés cherchant à approfondir leurs connaissances en électronique et mécanique.
Vous y trouverez des dizaines de chapitres captivants, allant de la conception du cadre à l'assemblage des moteurs, en passant par les systèmes de contrôle, l'alimentation et bien d'autres applications pratiques pour faire fonctionner votre hoverboard.
Chaque étape est expliquée en détail, avec des schémas clairs et des explications accessibles pour comprendre non seulement le fonctionnement de votre hoverboard, mais aussi les principes fondamentaux qui régissent la conception et l'assemblage de ce véhicule électrique.
🛡️ Paiement 100% sécurisé 🛡️
L’achat de votre livre ici est totalement sécurisé. Toutes les transactions sont protégées par un système de cryptage avancé, garantissant la confidentialité de vos informations. Aucune donnée bancaire n’est stockée ni partagée.
INTRODUCTION : L’AVENTURE COMMENCE !
🚀 Pourquoi construire un hoverboard toi-même ?
-
Découvrez les avantages de la fabrication maison.
-
Apprends comment fonctionne un hoverboard.
-
Personnalise ton propre modèle selon tes envies.
🏆 Ce que tu seras capable de faire après ce livre
-
Comprendre le fonctionnement d’un hoverboard.
-
Concevoir et assembler un modèle fonctionnel.
-
Programmer et tester les différentes fonctionnalités.
-
Ajouter des améliorations et résoudre les problèmes courants.
🔧 Les outils et composants indispensables
-
Liste détaillée des outils nécessaires (fer à souder, multimètre, tournevis, etc.).
-
Composants électroniques et mécaniques requis (batterie, moteurs, microcontrôleur, capteurs, etc.).
-
Équipements de sécurité recommandés.
CHAPITRE 1 : COMMENT MARCHE UN HOVERBOARD ?
🛸 Les bases de l’équilibre : comment ça tient debout ?
-
Principe de l’auto-équilibrage.
-
Rôle des capteurs et de l’algorithme de stabilisation.
⚙️ Les éléments essentiels : moteurs, capteurs, batteries
-
Explication du fonctionnement des moteurs brushless.
-
Présentation des capteurs gyroscopiques et accéléromètres.
-
Importance d’une batterie performante.
🎮 Le rôle du microcontrôleur : le cerveau de la machine
-
Les différents microcontrôleurs adaptés (Arduino, ESP32, STM32, etc.).
-
Comment ils interprètent les données des capteurs.
-
Communication entre les composants électroniques.
CHAPITRE 2 : CHOISIR LES BONS COMPOSANTS
🔋 Quelle batterie pour une bonne autonomie et sécurité ?
-
Types de batteries recommandées (Li-Ion, LiFePO4, etc.).
-
Capacité et tensions idéales pour une autonomie optimale.
-
Précautions de sécurité et entretien.
🚀 Moteurs : puissance, vitesse et compatibilité
-
Choisir les bons moteurs pour ton projet.
-
Différence entre moteurs à capteurs et sans capteurs.
-
Facteurs influençant la vitesse et la puissance.
🏗️ Châssis et roues : légèreté ET solidité
-
Matériaux recommandés pour le châssis.
-
Dimensions et types de roues adaptées.
-
Importance de la répartition du poids.
CHAPITRE 3 : LE CERVEAU DE L’HOVERBOARD – PROGRAMMATION FACILE !
🧠 Présentation du microcontrôleur
-
Comparatif entre Arduino, ESP32 et autres options.
-
Pourquoi le choix du microcontrôleur est crucial ?
💻 Écrire ton premier programme sans être un geek
-
Explication simple du langage de programmation (Arduino IDE, Python, C++).
-
Écriture d’un code basique pour tester le microcontrôleur.
🔌 Comment connecter capteurs et moteurs sans court-circuiter !
-
Guide de branchement sécurisé.
-
Protection contre les surtensions et courts-circuits.
-
Vérification et tests des connexions.
CHAPITRE 4 : ÉLECTRONIQUE ET CÂBLAGE – PAS À PAS
⚡ Comprendre le schéma électrique de ton hoverboard
-
Explication du circuit électrique général.
-
Comment distribuer l’énergie de manière efficace ?
🛠️ Branchements : comment tout connecter proprement
-
Organisation des fils et connexions.
-
Utilisation de connecteurs pour un montage modulable.
🔍 Éviter les erreurs classiques (et les explosions 💥)
-
Les erreurs fréquentes et comment les éviter.
-
Tests préliminaires avant la mise sous tension.
CHAPITRE 5 : LES CAPTEURS QUI FONT TENIR L’HOVERBOARD EN ÉQUILIBRE
🌀 Accéléromètre et gyroscope : comment ça marche ?
-
Fonctionnement des capteurs inertiels.
-
Intégration des données pour assurer la stabilité.
🔄 Convertir les mouvements en commandes précises
-
Algorithmes de stabilisation.
-
Réglage des paramètres pour un contrôle optimal.
🎛️ Tester les capteurs avant de tout assembler
-
Calibration des capteurs.
-
Vérification des données en temps réel.
CHAPITRE 6 : L’ASSEMBLAGE MÉCANIQUE – DONNE LUI UNE FORME !
🏗️ Construire un châssis solide et stylé
-
Matériaux et plans de construction.
-
Ajustements pour renforcer la structure.
🔩 Fixer les moteurs et les roues correctement
-
Montage sécurisé des composants mécaniques.
-
Tests pour garantir une bonne rotation des roues.
🎨 Personnalisation : LED, peinture, design unique
-
Ajout d’effets lumineux et sonores.
-
Peinture et finitions pour un look unique.
CHAPITRE 7 : TESTER ET AJUSTER TON HOVERBOARD
✅ Premiers tests en toute sécurité
-
Vérifications avant la mise en marche.
-
Comment éviter les accidents ?
🔧 Régler la vitesse et la réactivité
-
Ajustements logiciels pour une meilleure conduite.
-
Calibration des capteurs et du moteur.
🚀 Améliorer l’équilibre et la fluidité de conduite
-
Optimisation des algorithmes.
-
Tests pratiques pour un déplacement fluide.
CHAPITRE 8 : AJOUTER DES FONCTIONNALITÉS AVANCÉES
📲 Contrôle Bluetooth : piloter avec ton smartphone !
-
Intégration d’un module Bluetooth.
-
Création d’une application de pilotage.
🌟 Effets lumineux et sons personnalisés
-
Programmation des effets lumineux LED.
-
Ajout de sons interactifs.
⚙️ Ajout d’un écran pour afficher vitesse et batterie
-
Interface utilisateur simple et efficace.
-
Éléments à afficher pour une meilleure expérience.
CHAPITRE 9 : PROBLÈMES ET SOLUTIONS – RÉPARE COMME UN PRO !
🚨 Pourquoi mon hoverboard ne démarre pas ?
-
Diagnostic des pannes courantes.
-
Solutions rapides et efficaces.
🔥 Que faire si ça surchauffe ou si ça vibre ?
-
Analyse des causes et correctifs.
-
Améliorer le refroidissement et la stabilité.
🏁 Optimiser la durée de vie des composants
-
Entretien et maintenance réguliers.
-
Prolonger la durée de vie des batteries et moteurs.
CHAPITRE 10 : ALLER PLUS LOIN – CRÉE DES VERSIONS AMÉLIORÉES !
⚡ Hoverboard tout-terrain : booste la puissance !
-
Modifications pour une meilleure performance.
-
Adaptation aux terrains accidentés.
🚀 Transformer ton hoverboard en kart électrique
-
Ajout d’un siège et de commandes alternatives.
-
Modification des roues pour plus de stabilité.
🏆 Construire un modèle plus rapide et plus intelligent
-
Intégration de l’IA pour une meilleure navigation.
-
Ajout de nouvelles fonctionnalités innovantes.
23 comments