3d Game Engine In Python

Introduction
A 3D game leverages three-dimensional graphics to create immersive and interactive virtual worlds, enhancing realism and depth beyond what is possible in 2D games. In these games, environments and characters are rendered with three-dimensional models made up of polygons and textures, allowing for a more lifelike and engaging experience.
Unlike 2D games, where movement and interaction are confined to a flat plane, 3D games enable players to navigate through intricate spaces, perform complex tasks, solve puzzles, and engage in dynamic combat scenarios. This depth and freedom of movement contribute to a richer gameplay experience, exemplified by popular titles such as “Grand Theft Auto,” “The Legend of Zelda: Breath of the Wild,” and “The Witcher 3: Wild Hunt.


How To Code a 3D Game With Python?
To code a 3D game with Python, you will need to use a 3D game engine or library. Python, while traditionally known for its 2D game development, has several powerful tools for creating 3D environments. Here’s a general approach to get started:
Choose a 3D Game Engine or Library: Begin by selecting a 3D engine or library compatible with Python. Options like Pygame combined with OpenGL, Panda3D, or Godot with its Python API provide the necessary functionality to create and manage 3D graphics and game logic.
Set Up the Development Environment: Install the chosen engine or library along with any required dependencies. For instance, if you decide to use Panda3D, you will need to install the Panda3D engine and configure your project environment accordingly.
Create 3D Models and Assets: Design or acquire 3D models, textures, and other assets for your game. Tools such as Blender or Maya can be used to create these models, which will then need to be imported into your game engine.
Develop the Game Logic: Write scripts to handle the core mechanics of your game. This includes coding player controls, object interactions, and overall game rules. This step involves defining how objects move and interact within the 3D world.
Render the 3D World: Use the engine’s API to render your 3D scenes. This involves setting up cameras, lights, and rendering objects to create the visual experience of your game.
Implement Physics and Collision Detection: Integrate physics engines or write custom code to manage collisions and interactions between objects. This ensures realistic movement and interactions within your game world.
Test and Debug: Continuously test your game to ensure it runs smoothly. Debug any issues that arise and optimize performance to enhance the player experience.
Build and Distribute: Once your game is complete, prepare it for distribution. This may involve creating executable files or preparing the game for various platforms, depending on the tools used.

import pygame
pygame.init()
# Set up the display
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("My 3D Game")
# Main game loop
running = True
while running:
# Handle events
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Clear the screen
screen.fill((0, 0, 0)) # Fill with black color
# Update game state
# Render graphics
# Update the display
pygame.display.flip()
pygame.quit()
The code provided is a basic structure for setting up a game using Pygame, a popular Python library for creating games. Here’s a breakdown of what each part does:
Initialize Pygame:
pygame.init()
initializes all the Pygame modules required for the game. This must be called before using any other Pygame functionality.Set Up the Display: The
pygame.display.set_mode((width, height))
function creates the game window with the specified width and height (800×600 pixels in this case).pygame.display.set_caption("My 3D Game")
sets the window’s title to “My 3D Game”.Main Game Loop: The
while running:
loop represents the main game loop, which continually runs until the game is closed. This loop handles various aspects of the game:- Handle Events: The
for event in pygame.event.get():
loop processes events such as keyboard presses or mouse clicks. Theif event.type == pygame.QUIT:
condition checks if the window close button is clicked and setsrunning
toFalse
to exit the loop. - Clear the Screen:
screen.fill((0, 0, 0))
fills the entire screen with a black color. This effectively clears the previous frame, preparing it for the new frame. - Update Game State: This section (commented as
# Update game state
) is where game logic would be implemented, such as player movements, collisions, and other game dynamics. - Render Graphics: This section (commented as
# Render graphics
) is where you would draw game objects onto the screen. - Update the Display:
pygame.display.flip()
updates the full display Surface to the screen. This is necessary to reflect any changes made during the current loop iteration.
- Handle Events: The
Quit Pygame:
pygame.quit()
is called after the game loop exits to clean up and close all Pygame resources properly.

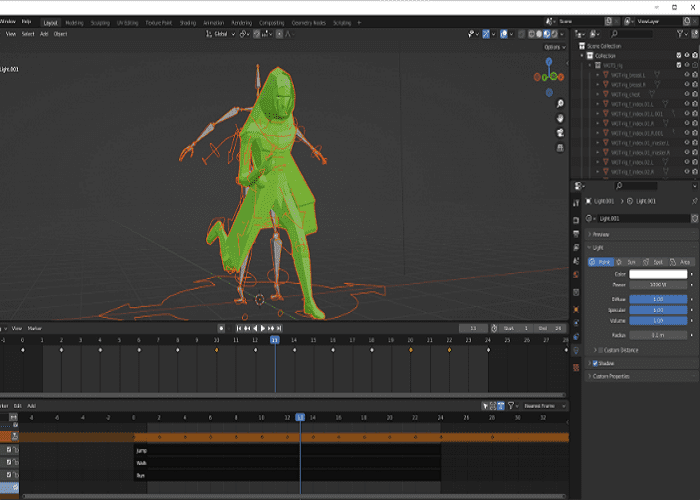
Panda3D
Panda3D is a powerful and flexible open-source game engine designed for creating 3D games and simulations. Developed by Disney and maintained by the community, Panda3D provides a comprehensive set of tools for rendering 3D graphics, managing physics, and handling complex game logic. Its features include support for advanced graphics techniques like shaders and lighting, robust scene graph management for organizing game objects, and an easy-to-use Python API for scripting game behavior.
One of the key strengths of Panda3D is its integration with Python, making it accessible to both experienced developers and those new to game programming. The engine allows developers to leverage Python’s simplicity and readability to create intricate 3D worlds and interactive experiences without having to delve deeply into lower-level programming languages. Panda3D’s extensive documentation and active community contribute to a supportive development environment, providing resources and assistance for overcoming challenges in game design and implementation.
Whether you’re developing a complex simulation, an immersive game, or a virtual reality experience, Panda3D offers a versatile platform to bring your creative vision to life. Its combination of powerful features and user-friendly Python integration makes it a valuable tool for 3D game development.
How To Use Panda3D?
To use Panda3D, start by installing it either via pip with pip install panda3d
or by downloading the SDK from the official website. Set up your development environment by creating a project directory and writing a basic Python script to initialize a window and render a simple scene.
Explore the extensive Panda3D documentation and tutorials to understand the API and learn how to implement features. Develop your game by creating 3D models with tools like Blender, scripting game logic, and designing scenes. Regularly test your game to identify and fix issues, then package it for distribution using Panda3D’s tools. This process allows you to build and share sophisticated 3D applications and games using Panda3D.

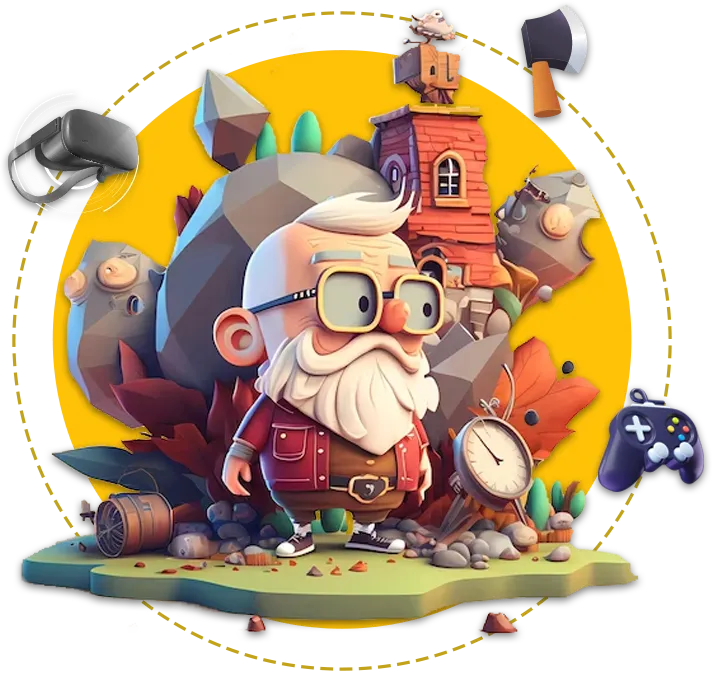
First 3D Game
from panda3d.core import *
from direct.showbase.ShowBase import ShowBase
class MyGame(ShowBase):
def __init__(self):
ShowBase.__init__(self)
# Load the environment
self.environ = self.loader.loadModel("models/environment")
self.environ.reparentTo(self.render)
self.environ.setScale(0.25, 0.25, 0.25)
self.environ.setPos(-8, 42, 0)
# Load the player model
self.player = self.loader.loadModel("models/panda")
self.player.reparentTo(self.render)
self.player.setScale(0.005)
self.player.setPos(0, 0, 0)
# Set up controls
self.accept("arrow_left", self.move, ["left"])
self.accept("arrow_right", self.move, ["right"])
self.accept("arrow_up", self.move, ["forward"])
self.accept("arrow_down", self.move, ["backward"])
def move(self, direction):
if direction == "left":
self.player.setX(self.player.getX() - 1)
elif direction == "right":
self.player.setX(self.player.getX() + 1)
elif direction == "forward":
self.player.setY(self.player.getY() + 1)
elif direction == "backward":
self.player.setY(self.player.getY() - 1)
if __name__ == "__main__":
app = MyGame()
app.run()
The code snippet sets up a basic 3D game using Panda3D. It defines a MyGame
class that inherits from ShowBase
, which is the core class for rendering and handling user input in Panda3D. In the __init__
method, the game initializes the environment by loading a model ("models/environment"
) and a player model ("models/panda"
). The environment is scaled and positioned, while the player is scaled down and placed at the origin.
The code sets up basic controls for moving the player model using the arrow keys. The self.accept
method binds the arrow keys to the move
method, which updates the player’s position based on the direction of movement. For instance, pressing the left arrow key moves the player left along the X-axis, while pressing the up arrow key moves the player forward along the Y-axis.
The move
method adjusts the player’s position by changing its X or Y coordinates depending on the direction specified. Finally, the app.run()
call starts the Panda3D application, allowing the game to run and respond to user inputs.

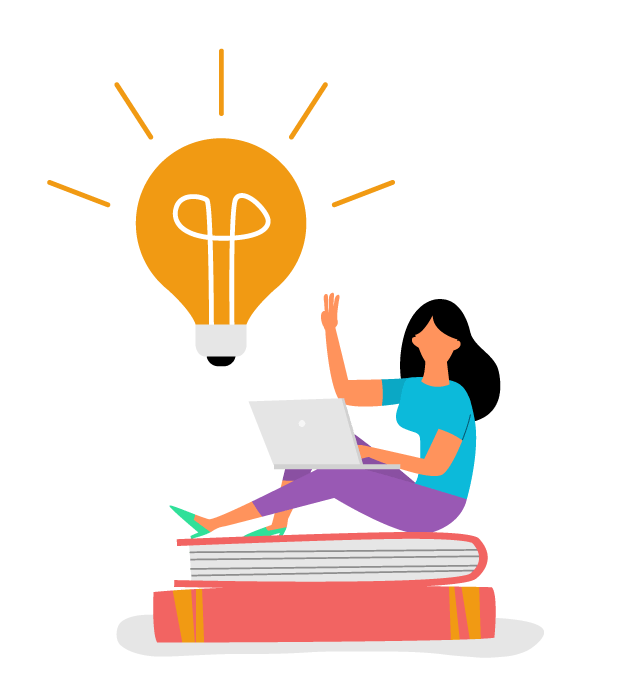
Summary
Creating a 3D game involves understanding and implementing a range of concepts and tools to build immersive and interactive experiences. The journey starts with setting up a 3D environment and loading models that bring the virtual world to life. Key aspects include managing object placement, implementing user controls, and handling real-time interactions within the 3D space.
By leveraging powerful game engines like Panda3D, developers can efficiently create and manipulate 3D environments, making it easier to focus on the creative and gameplay aspects of game development. The ability to control and render 3D models, along with managing game physics and interactions, is crucial for building engaging and visually compelling games.
In summary, developing a 3D game requires a combination of technical knowledge and creative design, utilizing specialized tools and libraries to bring complex, interactive worlds to life.
Post Comment