Jumping Game In Python

Introduction
In this game, players control a character that must jump to overcome obstacles, reach platforms, and navigate various challenges. Using libraries like Pygame, you can create an engaging and interactive experience where timing and precision are crucial.
The game involves implementing mechanics such as gravity, jumping physics, and collision detection to create a dynamic and fun environment. Players will need to master their timing to jump accurately, avoid hazards, and achieve high scores. This project not only offers an exciting gameplay experience but also serves as a practical exercise in game development, physics simulation, and user input handling.
Coding a Juming Game in Python
Coding a jumping game in Python typically involves creating a simple platformer where a character must navigate through obstacles and platforms by jumping. Using libraries like Pygame
, you can implement essential features such as gravity, collision detection, and responsive controls to make the game engaging.
The basic steps include setting up a game window, handling user inputs to control the character’s movement and jumping actions, and applying physics to simulate realistic jumps and falls. You’ll also need to manage the game state, including scoring and level progression, while ensuring smooth graphics rendering and responsive gameplay. This project not only provides a fun introduction to game development but also allows you to explore concepts such as sprite animation, physics simulation, and real-time interaction in Python.

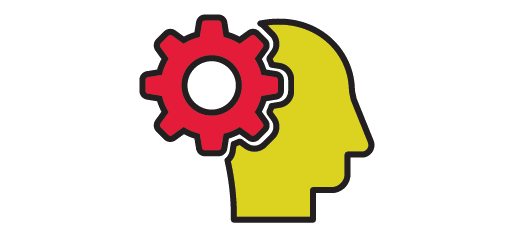
What You Need To Know
To successfully code a jumping game in Python, you’ll need to grasp several key concepts and skills:
Python Programming Basics: Familiarity with Python syntax and core programming concepts, including variables, control flow, and functions.
Game Development Fundamentals: Understanding of basic game development principles, such as game loops, event handling, and game state management.
Pygame Library: Proficiency with
Pygame
for creating game windows, handling graphics, and managing user input. Key functions include initializing Pygame, loading and displaying images, and capturing keyboard events.Physics Simulation: Knowledge of basic physics principles to simulate gravity, jumping mechanics, and collision detection. You’ll need to calculate how objects move and interact under various forces.
Collision Detection: Ability to implement logic for detecting when the player character collides with platforms or other obstacles. This involves checking for overlaps between sprites.
Animation and Drawing: Skills in rendering and animating sprites, including drawing characters, platforms, and other game elements on the screen.
User Input Handling: Managing keyboard input to control the character’s movements, such as jumping and running.
Game State Management: Keeping track of various game states, such as player health, score, and level progression.
Mastering these elements will enable you to build a functional and enjoyable jumping game, offering a solid foundation for more complex game development projects.
Move Right, Move Left, Jump!
Imagine the thrill of controlling a character as it leaps through a colorful world, navigating obstacles and reaching new heights. That’s the essence of a jumping game! And you might be surprised to learn that creating one is simpler than you think. In a jumping game, the mechanics are straightforward: you can move your character left or right, and you can make them jump.
That’s it! But don’t let the simplicity fool you – the fun is in the challenge and the sense of achievement as you conquer each level. Here’s why coding a jumping game is a blast: Quick and Easy to Learn: With just a few basic programming concepts under your belt, you can start coding your own jumping game.
The mechanics are simple, making it an excellent choice for beginners. Instant Gratification: There’s something incredibly satisfying about seeing your character jump, dodge obstacles, and reach new heights – all controlled by your code! Endless Creativity: While the core mechanics are simple, the possibilities for creativity are endless. You can design imaginative levels, create unique characters, and add exciting challenges to keep players engaged.
Accessible to Everyone: Whether you’re a seasoned coder or just starting out, you can create a jumping game. With the wealth of tutorials, resources, and game development tools available online, anyone can dive in and start creating.
Addictive Gameplay: The simple yet addictive gameplay of jumping games keeps players coming back for more. Whether you’re testing your skills to beat your high score or challenging friends to see who can reach the highest level, there’s always something to strive for.
Share and Connect: Once you’ve created your jumping game, you can share it with others and connect with fellow game developers. Whether it’s sharing your game on social media, participating in game jams, or joining online communities, there’s a whole world of sharing and collaboration waiting for you.

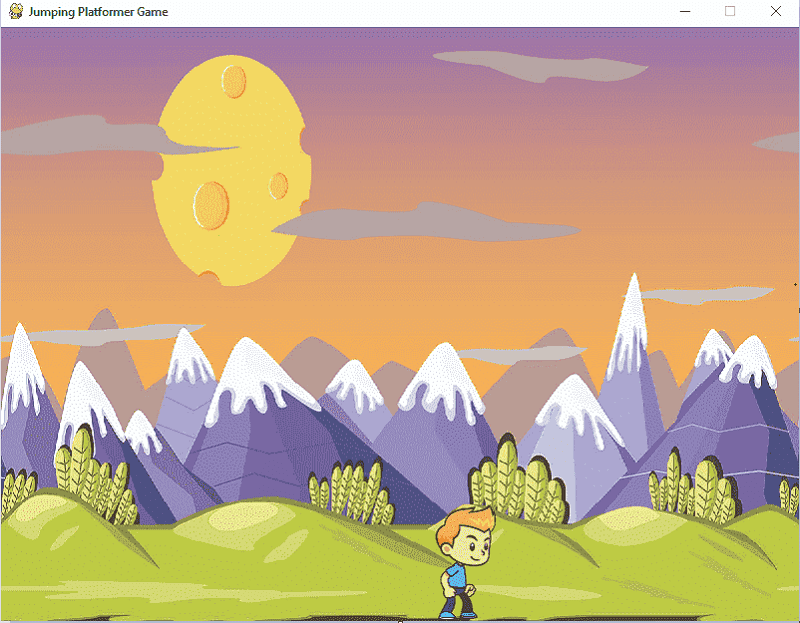
Jumping Game With Python (Full Code)
# Import pygame module
import pygame
# Import sys module
import sys
# Initialize Pygame
pygame.init()
# Set up the screen
# Define screen width and height
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
# Create a display surface with defined dimensions
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
# Set the caption of the window
pygame.display.set_caption("Jumping Platformer Game")
# Load images
# Load the player image when facing right and convert it to alpha format
player_img_right = pygame.image.load("player_right.png").convert_alpha()
# Load the player image when facing left and convert it to alpha format
player_img_left = pygame.image.load("player_left.png").convert_alpha()
# Load the player image when jumping and convert it to alpha format
player_img_jump = pygame.image.load("player_jump.png").convert_alpha()
# Load the background image
background_img = pygame.image.load("background.png").convert()
# Scale background image to fit the screen
# Scale the background image to match the screen dimensions
background_img = pygame.transform.scale(background_img, (SCREEN_WIDTH, SCREEN_HEIGHT))
# Get player image dimensions
# Get the width and height of the player image when facing right
player_width, player_height = player_img_right.get_size()
# Player attributes
# Set the initial x position of the player at the center of the screen
player_x = SCREEN_WIDTH // 2 - player_width // 2
# Set the initial y position of the player at the bottom of the screen
player_y = SCREEN_HEIGHT - player_height
# Set the speed of the player movement
player_speed = 5
# Set the initial jump force of the player
jump_force = -17
# Set the gravity force acting on the player
gravity = 0.5
# Set a flag indicating whether the player is on the ground
on_ground = True
# Set a flag indicating whether the player is currently jumping
jumping = False
# Set the initial player image to face right
player_image = player_img_right
# Function to draw player
# Draw the player on the screen
def draw_player():
# Blit the player image onto the screen at the specified position
screen.blit(player_image, (player_x, player_y))
# Function to handle player movement
# Update the position and state of the player based on user input
def move_player():
# Access global variables to update their values
global player_x, player_y, on_ground, jumping, jump_force
# Get the state of the keyboard
keys = pygame.key.get_pressed()
# Move the player to the left if the left arrow key is pressed
if keys[pygame.K_LEFT] and player_x > 0:
player_x -= player_speed
# Check if the player is still on the ground and not at the bottom of the screen
if player_y + player_height < SCREEN_HEIGHT:
on_ground = False
# Move the player to the right if the right arrow key is pressed
if keys[pygame.K_RIGHT] and player_x < SCREEN_WIDTH - player_width:
player_x += player_speed
# Check if the player is still on the ground and not at the bottom of the screen
if player_y + player_height < SCREEN_HEIGHT:
on_ground = False
# Jumping mechanic
# Execute if the player is currently jumping
if jumping:
# Update the y position of the player based on the jump force
player_y += jump_force
# Increase the jump force by the gravity
jump_force += gravity
# Check if the player has reached the ground
if player_y >= SCREEN_HEIGHT - player_height:
# Reset the y position of the player to be on the ground
player_y = SCREEN_HEIGHT - player_height
# Reset the jump force
jump_force = -17
# Set the on_ground flag to True
on_ground = True
# Set the jumping flag to False
jumping = False
# Main game loop
# Create a clock object to control the frame rate
clock = pygame.time.Clock()
# Enter the main game loop
while True:
# Event handling
# Iterate over each event in the event queue
for event in pygame.event.get():
# Check if the user has clicked the close button
if event.type == pygame.QUIT:
# Quit Pygame
pygame.quit()
# Exit the program
sys.exit()
# Check if the user has pressed a key
elif event.type == pygame.KEYDOWN:
# Check if the space key is pressed and the player is on the ground
if event.key == pygame.K_SPACE and on_ground:
# Set the jumping flag to True
jumping = True
# Set the on_ground flag to False
on_ground = False
# Check if the left arrow key is pressed and the player is on the ground
elif event.key == pygame.K_LEFT and on_ground:
# Change the player image to face left
player_image = player_img_left
# Check if the right arrow key is pressed and the player is on the ground
elif event.key == pygame.K_RIGHT and on_ground:
# Change the player image to face right
player_image = player_img_right
# Move player
# Update the player's position and state
move_player()
# Draw background
# Blit the background image onto the screen at position (0, 0)
screen.blit(background_img, (0, 0))
# Draw player
# Call the draw_player function to display the player on the screen
draw_player()
# Update the display
# Flip the display to show the changes
pygame.display.flip()
# Set the frame rate
# Limit the frame rate to 60 frames per second
clock.tick(60)
Now it’s time to see your jumping game in action! To execute the code and see how it looks, follow these steps:
Open PyCharm: Ensure that PyCharm is installed and set up on your computer.
Create a New Project: If you haven’t already, create a new Python project in PyCharm.
Add a New Python File: Inside your project, add a new Python file where you will paste the code.
Copy and Paste the Code: Copy the provided code and paste it into your new Python file.
Run the Code: Click the “Run” button in PyCharm to execute the code.
If everything is set up correctly, you should see the jumping game display on your screen, allowing you to control the character and experience the gameplay. If you encounter any issues, make sure all necessary libraries are installed and review the code for any errors. Enjoy building and playing your jumping game!

Main Algorithms To Understand
Setting Up The Screen:
import pygame
import sys
# Initialize Pygame
pygame.init()
# Set up the screen
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("Jumping Platformer Game")
To set up the screen for your jumping platformer game using Pygame
, you’ll start by initializing the Pygame library with pygame.init()
, which prepares all necessary modules for game development. Next, define the dimensions of your game window by specifying the width and height, such as 800 pixels by 600 pixels.
Using pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
, create the game window with these dimensions, which will serve as the main canvas for your game. Finally, set the title of your window with pygame.display.set_caption("Jumping Platformer Game")
to give your game a distinctive name on the window’s title bar. This setup forms the foundation of your game’s graphical interface, ready for further development of game mechanics and features.

Load Images:
# Load images
player_img_right = pygame.image.load("player_right.png").convert_alpha()
player_img_left = pygame.image.load("player_left.png").convert_alpha()
player_img_jump = pygame.image.load("player_jump.png").convert_alpha()
background_img = pygame.image.load("background.png").convert()
To enhance your jumping game, you’ll need to load various images for the game elements. Start by loading the images using pygame.image.load()
, which reads the image files from your project directory. For example, player_img_right
, player_img_left
, and player_img_jump
represent the different states of the player character—facing right, facing left, and jumping, respectively.
These images are loaded with convert_alpha()
to handle transparency, ensuring smooth rendering. Additionally, load the background image with background_img = pygame.image.load("background.png").convert()
, which provides the game’s backdrop. This background image is loaded without transparency handling as it is not necessary for backgrounds. These images will be used throughout the game to visually represent the player’s actions and the game environment, making the gameplay experience more immersive and engaging.

Get Player Image Dimension:
player_width, player_height = player_img_right.get_size()
This line of code resizes the background image to fit the dimensions of the game screen. The pygame.transform.scale() function is used to scale the image to the specified width and height, which are set to SCREEN_WIDTH and SCREEN_HEIGHT respectively. This ensures that the background image covers the entire game screen.

Player Attributes:
# Player attributes
player_x = SCREEN_WIDTH // 2 - player_width // 2
player_y = SCREEN_HEIGHT - player_height
player_speed = 5
jump_force = -17
gravity = 0.5
on_ground = True
jumping = False
player_image = player_img_right # Initially facing right
The player attributes section sets up the initial properties and position of the player character in the game. Here, player_x
and player_y
determine the starting position of the player by centering it horizontally and placing it at the bottom of the screen. player_speed
controls how fast the player moves left or right. jump_force
defines the initial force applied when the player jumps, creating an upward motion, while gravity
gradually pulls the player back down, simulating realistic jumping physics.
The on_ground
variable checks if the player is on the ground to enable or disable jumping, and jumping
keeps track of whether the player is currently in the air. Finally, player_image
is set to player_img_right
, indicating that the player initially faces right. These attributes are essential for managing player movement and interaction within the game.

Function to Draw Player:
# Function to draw player
def draw_player():
screen.blit(player_image, (player_x, player_y))
This function is responsible for drawing the player on the screen. It uses the blit method of the screen surface to draw the player image (player_image) at the current position (player_x, player_y).
The player_x and player_y variables determine the position of the player on the screen, and player_image contains the image of the player to be drawn.

Function to Handle Player Movement:
# Function to handle player movement
def move_player():
global player_x, player_y, on_ground, jumping, jump_force
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and player_x > 0:
player_x -= player_speed
if player_y + player_height < SCREEN_HEIGHT:
on_ground = False
if keys[pygame.K_RIGHT] and player_x < SCREEN_WIDTH - player_width:
player_x += player_speed
if player_y + player_height < SCREEN_HEIGHT:
on_ground = False
# Jumping mechanic
if jumping:
player_y += jump_force
jump_force += gravity
if player_y >= SCREEN_HEIGHT - player_height:
player_y = SCREEN_HEIGHT - player_height
jump_force = -17
on_ground = True
jumping = False
The move_player
function controls the movement of the player character, including horizontal movement and jumping mechanics. It starts by accessing the global variables related to the player’s position and state. The function checks for key presses using pygame.key.get_pressed()
. If the left arrow key is pressed and the player isn’t at the screen’s left edge, the player’s x-coordinate is decreased, moving them left. Similarly, if the right arrow key is pressed and the player isn’t at the screen’s right edge, the x-coordinate is increased, moving them right.
For jumping, if the jumping
flag is set, the player’s y-coordinate is adjusted by jump_force
, which decreases over time due to gravity, simulating a jump and fall effect. When the player lands back on the ground, indicated by reaching the bottom of the screen, the player’s y-coordinate is reset, the jump force is reinitialized, and the on_ground
and jumping
flags are updated accordingly. This function ensures the player character moves correctly and interacts with the game environment as expected.

Function to Draw Player: Main Loop
# Main game loop
clock = pygame.time.Clock()
while True:
# Event handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE and on_ground:
jumping = True
on_ground = False
elif event.key == pygame.K_LEFT and on_ground:
player_image = player_img_left
elif event.key == pygame.K_RIGHT and on_ground:
player_image = player_img_right
# Move player
move_player()
# Draw background
screen.blit(background_img, (0, 0))
# Draw player
draw_player()
pygame.display.flip()
# Set the frame rate
clock.tick(60)
The main game loop drives the continuous execution of the game, handling events, updating the game state, and rendering the game graphics. It begins by creating a Clock
object to manage the frame rate. Within the loop, it processes events by checking if the player has requested to quit the game (pygame.QUIT
) or pressed specific keys. For instance, pressing the space bar initiates a jump if the player is on the ground, and pressing the left or right arrow keys changes the player’s image to face the corresponding direction, provided the player is also on the ground.
After handling events, the move_player
function is called to update the player’s position based on input and physics. The background image is drawn on the screen, followed by the player’s image, using screen.blit()
for rendering. The pygame.display.flip()
function updates the display to show the latest changes. Finally, clock.tick(60)
ensures that the game runs at a consistent frame rate of 60 frames per second, providing smooth gameplay. This loop runs continuously, enabling real-time interaction and animation in the game.

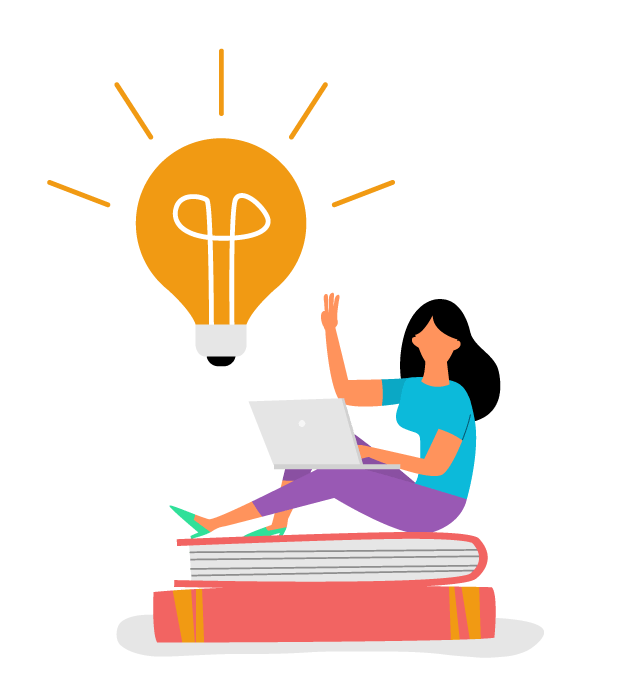
Summary
In summary, this code snippet represents a fundamental jumping game implemented using Pygame. The setup involves initializing Pygame, creating a game window, and loading images for the player and background. The player’s movement is managed through the move_player
function, which handles horizontal movement and jumping mechanics based on user input and game physics.
The main game loop continuously processes events, updates the game state, and renders the graphics. Key features include handling player input for jumping and movement, updating the player’s position, and ensuring smooth animation with a consistent frame rate. Overall, this setup provides a basic but functional framework for a jumping game, allowing for further development and customization.
Post Comment