Password Brute-Force In Python
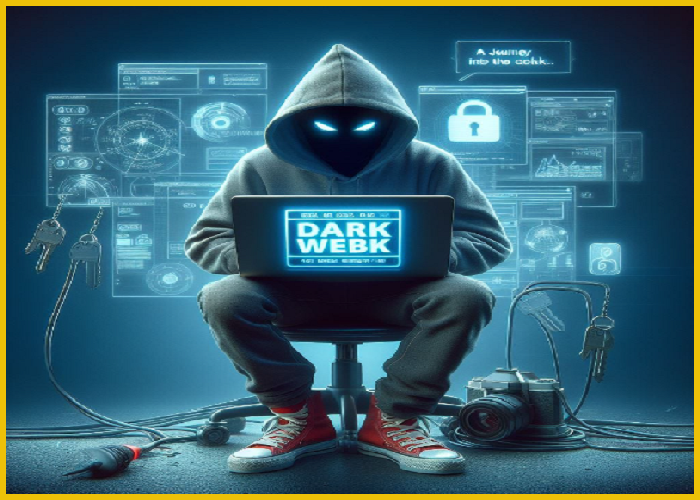
Introduction
In the world of cybersecurity, password cracking is a critical topic. It involves attempting to break into a system or an account by systematically trying all possible passwords until the correct one is found. Brute-force attacks, though straightforward in concept, are often used to test the strength of passwords and to identify weaknesses in security measures.
Understanding Brute Force
Brute-force attacks involve trying every possible combination of characters until the correct password is discovered. This method does not rely on any specific knowledge about the target apart from the format and length of the password.
Implementing a Brute-Force Password Cracker in Python
In this tutorial, we’ll walk through the process of creating a basic brute-force password cracker using Python. We’ll focus on a scenario where the password consists of lowercase letters (a-z) and digits (0-9) and is of a fixed length.
Step 1: Generating Password Combinations
The first step is to generate all possible combinations of characters that could form a password of a given length. We’ll use Python’s itertools
module for this purpose, specifically the product
function.
Step 2: Testing Each Combination
In the brute_force_password
function, our goal is to systematically generate all possible combinations of characters up to a specified length (password_length
) using the characters provided in possible_chars
. Each generated password (attempt_str
) is then tested against a target password (for demonstration purposes, we’ll use ‘abcd’).
Detailed Implementation
Here’s how we can develop this step further:
Explanation:
Outer Loop (
for length in range(1, password_length + 1):
):- This loop controls the length of the passwords we attempt to crack. It iterates from 1 up to
password_length
, which specifies the maximum length of the password we’re trying to guess.
- This loop controls the length of the passwords we attempt to crack. It iterates from 1 up to
Inner Loop (
for attempt in itertools.product(possible_chars, repeat=length):
):- For each length specified by the outer loop, we use
itertools.product
to generate all possible combinations of characters frompossible_chars
of the current length (length
). itertools.product
generates tuples containing characters frompossible_chars
, repeatedlength
times. For example, iflength
is 2 andpossible_chars
is'abc'
, it will generate tuples like('a', 'a'), ('a', 'b'), ('a', 'c'), ('b', 'a'), ..., ('c', 'c')
.
- For each length specified by the outer loop, we use
Generating Password Attempts:
- Each tuple (
attempt
) generated byitertools.product
is joined into a string (attempt_str
) representing a potential password.
- Each tuple (
Password Checking:
- In a real-world scenario, you would compare
attempt_str
against a target password stored in a database or another secure location. For demonstration, we compare it against the string'abcd'
. - If
attempt_str
matches the target password ('abcd'
in this case), we print a message indicating that the password has been cracked and return the password.
- In a real-world scenario, you would compare
Printing Attempts (Optional):
- For visibility and debugging purposes, each password attempt (
attempt_str
) can be printed or logged. This helps in understanding the progress of the brute-force attack.
- For visibility and debugging purposes, each password attempt (
Returning Results:
- If no password matches within the specified length and character set, the function returns a message indicating that the password was not found.
Considerations:
Performance: Brute-force attacks can be slow, especially for longer passwords or more complex character sets. Optimizations such as parallelization or smarter algorithms are necessary for practical use.
Ethical Considerations: Unauthorized access to systems or accounts is illegal and unethical. This script should only be used for educational purposes or with explicit permission from the system owner.
Step 3: Practical Usage and Considerations
While the example illustrates the basic mechanics of a brute-force attack, there are several practical and ethical considerations to keep in mind before attempting or deploying such code.
Performance Considerations
Brute-force attacks, by their nature, involve trying every possible combination of characters until the correct password is found. This process can be extremely time-consuming and resource-intensive, especially when dealing with:
- Password Length: The longer the password, the exponentially more combinations there are to try.
- Character Set: Including uppercase letters, special characters, and non-alphanumeric characters increases the complexity and number of possible combinations.
- Hardware Resources: Brute-forcing can require significant computational power, particularly for large character sets or long passwords.
To improve performance, strategies like parallelization (using multiple processors or threads) or optimizing the algorithm (reducing unnecessary computations) can be implemented. However, even with optimizations, brute-forcing strong passwords can still be impractical or infeasible.
Legal and Ethical Concerns
Brute-force attacks are typically considered unauthorized access under most jurisdictions and can have serious legal implications. It’s crucial to adhere to ethical guidelines and legal regulations:
- Unauthorized Access: Attempting to access systems, networks, or accounts without explicit permission is illegal in many jurisdictions and can lead to criminal charges.
- Ethical Considerations: Respect for privacy and the integrity of systems should guide any cybersecurity practice. Brute-forcing passwords without proper authorization violates these principles.
Responsible Use and Ethical Guidelines
When developing or using a brute-force password cracker:
- Educational Purposes: Use such tools and techniques solely for educational purposes to understand cybersecurity vulnerabilities and improve defenses.
- Penetration Testing: Conduct brute-force attacks only with explicit permission from the owner of the system or network as part of authorized penetration testing.
- Security Awareness: Promote strong password policies, multi-factor authentication (MFA), and other security measures to mitigate the risk of successful brute-force attacks.
While brute-force attacks serve as a fundamental method for testing password strength and understanding security vulnerabilities, they must be approached with caution and responsibility. Prioritize legal compliance, ethical standards, and respect for privacy when engaging in any form of cybersecurity research or practice.
By fostering a culture of security awareness and responsible usage of cybersecurity tools, we contribute to a safer digital environment where privacy and data integrity are protected. Always seek appropriate permissions and adhere to legal regulations to ensure ethical conduct in cybersecurity practices.
Post Comment