Python Scripts For Beginners: Cryptography
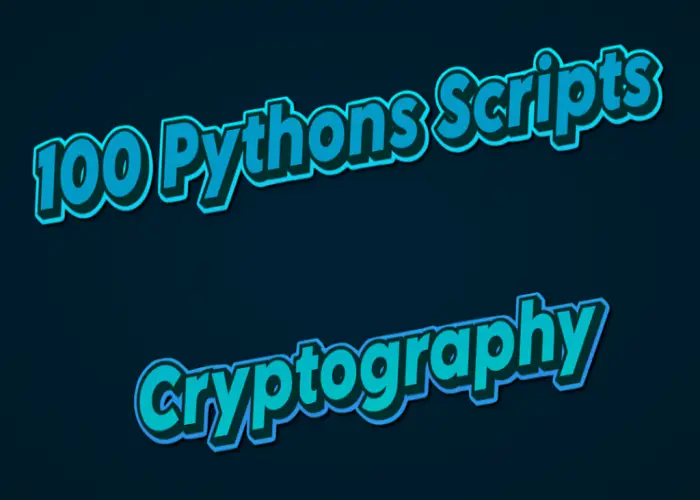
Collection of 100 Python scripts for Cryptography, each designed to help with fundamental tasks and provide useful examples
100 Python Scripts For Beginners: Cryptography
1. Read a CSV File
import pandas as pd
data = pd.read_csv('data.csv')
print(data)
Reads and prints a CSV file using pandas.
2. Write Data to a CSV File
import pandas as pd
data = {'Name': ['John', 'Anna'], 'Age': [28, 24]}
df = pd.DataFrame(data)
df.to_csv('output.csv', index=False)
Writes data to a CSV file.
3. Read an Excel File
import pandas as pd
data = pd.read_excel('data.xlsx')
print(data)
Reads and prints an Excel file.
4. Write Data to an Excel File
import pandas as pd
data = {'Name': ['John', 'Anna'], 'Age': [28, 24]}
df = pd.DataFrame(data)
df.to_excel('output.xlsx', index=False)
Writes data to an Excel file.
5. Read a JSON File
import json
with open('data.json', 'r') as file:
data = json.load(file)
print(data)
Reads and prints a JSON file.
6. Write Data to a JSON File
import json
data = {'Name': 'John', 'Age': 28}
with open('output.json', 'w') as file:
json.dump(data, file)
Writes data to a JSON file.
7. Read a Text File
with open('data.txt', 'r') as file:
content = file.read()
print(content)
Reads and prints a text file.
8. Write Data to a Text File
with open('output.txt', 'w') as file:
file.write("Hello, World!")
Writes data to a text file.
9. Append Data to a Text File
with open('output.txt', 'a') as file:
file.write("\nAppended Line")
Appends data to a text file.
10. Read Data from a Database (SQLite)
import sqlite3
conn = sqlite3.connect('database.db')
cursor = conn.cursor()
cursor.execute("SELECT * FROM table_name")
data = cursor.fetchall()
print(data)
conn.close()
Reads and prints data from an SQLite database.
11. Write Data to a Database (SQLite)
import sqlite3
conn = sqlite3.connect('database.db')
cursor = conn.cursor()
cursor.execute("CREATE TABLE IF NOT EXISTS table_name (id INTEGER PRIMARY KEY, name TEXT)")
cursor.execute("INSERT INTO table_name (name) VALUES ('John')")
conn.commit()
conn.close()
Writes data to an SQLite database.
12. Filter Data in a DataFrame
import pandas as pd
data = pd.read_csv('data.csv')
filtered_data = data[data['Age'] > 30]
print(filtered_data)
Filters rows in a DataFrame based on a condition.
13. Group Data in a DataFrame
import pandas as pd
data = pd.read_csv('data.csv')
grouped_data = data.groupby('Category').sum()
print(grouped_data)
Groups data in a DataFrame by a column and performs aggregation.
14. Merge Two DataFrames
import pandas as pd
df1 = pd.read_csv('data1.csv')
df2 = pd.read_csv('data2.csv')
merged_df = pd.merge(df1, df2, on='ID')
print(merged_df)
Merges two DataFrames based on a common column.
15. Pivot Table
import pandas as pd
data = pd.read_csv('data.csv')
pivot_table = data.pivot_table(values='Value', index='Date', columns='Category')
print(pivot_table)
Creates a pivot table from a DataFrame.
16. Drop Duplicate Rows
import pandas as pd
data = pd.read_csv('data.csv')
unique_data = data.drop_duplicates()
print(unique_data)
Drops duplicate rows from a DataFrame.
17. Fill Missing Values
import pandas as pd
data = pd.read_csv('data.csv')
filled_data = data.fillna(method='ffill')
print(filled_data)
Fills missing values in a DataFrame using forward fill.
18. Drop Rows with Missing Values
import pandas as pd
data = pd.read_csv('data.csv')
cleaned_data = data.dropna()
print(cleaned_data)
Drops rows with missing values from a DataFrame.
19. Save DataFrame to Parquet
import pandas as pd
data = pd.read_csv('data.csv')
data.to_parquet('data.parquet')
Saves a DataFrame to a Parquet file.
20. Read Parquet File
import pandas as pd
data = pd.read_parquet('data.parquet')
print(data)
Reads and prints data from a Parquet file.
21. Convert DataFrame to JSON
import pandas as pd
data = pd.read_csv('data.csv')
json_data = data.to_json()
print(json_data)
Converts a DataFrame to a JSON string.
22. Convert JSON to DataFrame
import pandas as pd
import json
with open('data.json', 'r') as file:
data = json.load(file)
df = pd.json_normalize(data)
print(df)
Converts JSON data to a DataFrame.
23. Calculate Statistics of a DataFrame
import pandas as pd
data = pd.read_csv('data.csv')
print(data.describe())
Calculates and prints basic statistics of a DataFrame.
24. Normalize Data
import pandas as pd
from sklearn.preprocessing import MinMaxScaler
data = pd.read_csv('data.csv')
scaler = MinMaxScaler()
normalized_data = scaler.fit_transform(data[['Value']])
print(normalized_data)
Normalizes data using Min-Max scaling.
25. Standardize Data
import pandas as pd
from sklearn.preprocessing import StandardScaler
data = pd.read_csv('data.csv')
scaler = StandardScaler()
standardized_data = scaler.fit_transform(data[['Value']])
print(standardized_data)
Standardizes data to have a mean of 0 and a standard deviation of 1.
26. Load Data from API
import requests
response = requests.get('https://api.example.com/data')
data = response.json()
print(data)
Fetches and prints data from an API.
27. Save DataFrame to SQL Database
import pandas as pd
import sqlite3
data = pd.read_csv('data.csv')
conn = sqlite3.connect('database.db')
data.to_sql('table_name', conn, if_exists='replace', index=False)
conn.close()
Saves a DataFrame to an SQL database.
28. Load DataFrame from SQL Database
import pandas as pd
import sqlite3
conn = sqlite3.connect('database.db')
data = pd.read_sql('SELECT * FROM table_name', conn)
print(data)
conn.close()
Loads data from an SQL database into a DataFrame.
29. Write Data to XML
import pandas as pd
data = pd.read_csv('data.csv')
data.to_xml('data.xml')
Writes a DataFrame to an XML file.
30. Read Data from XML
import pandas as pd
data = pd.read_xml('data.xml')
print(data)
Reads and prints data from an XML file.
31. Create a DataFrame from Scratch
import pandas as pd
data = {'Name': ['John', 'Anna'], 'Age': [28, 24]}
df = pd.DataFrame(data)
print(df)
Creates a DataFrame from scratch.
32. Flatten Nested JSON
import pandas as pd
import json
with open('nested_data.json', 'r') as file:
data = json.load(file)
df = pd.json_normalize(data, sep='_')
print(df)
Flattens nested JSON data into a DataFrame.
33. Merge DataFrames with Different Indexes
import pandas as pd
df1 = pd.DataFrame({'A': [1, 2]}, index=['a', 'b'])
df2 = pd.DataFrame({'B': [3, 4]}, index=['b', 'c'])
merged_df = df1.join(df2)
print(merged_df)
Merges DataFrames with different indexes.
34. Append Rows to DataFrame
import pandas as pd
data1 = pd.DataFrame({'Name': ['John'], 'Age': [28]})
data2 = pd.DataFrame({'Name': ['Anna'], 'Age': [24]})
appended_data = data1.append(data2, ignore_index=True)
print(appended_data)
Appends rows to a DataFrame.
35. Remove Columns from DataFrame
import pandas as pd
data = pd.read_csv('data.csv')
cleaned_data = data.drop(columns=['UnwantedColumn'])
print(cleaned_data)
Removes specified columns from a DataFrame.
36. Rename Columns in DataFrame
import pandas as pd
data = pd.read_csv('data.csv')
data.rename(columns={'OldName': 'NewName'}, inplace=True)
print(data)
Renames columns in a DataFrame.
37. Create DataFrame with MultiIndex
import pandas as pd
arrays = [['A', 'A', 'B', 'B'], [1, 2, 1, 2]]
index = pd.MultiIndex.from_arrays(arrays, names=('Letter', 'Number'))
data = pd.DataFrame({'Value': [10, 20, 30, 40]}, index=index)
print(data)
Creates a DataFrame with a MultiIndex.
38. Reshape DataFrame
import pandas as pd
data = pd.read_csv('data.csv')
reshaped_data = data.melt(id_vars=['ID'], value_vars=['Value1', 'Value2'])
print(reshaped_data)
Reshapes a DataFrame using melt
.
39. Pivot DataFrame
import pandas as pd
data = pd.read_csv('data.csv')
pivoted_data = data.pivot(index='ID', columns='Category', values='Value')
print(pivoted_data)
Creates a pivot table from a DataFrame.
40. Save DataFrame to HDF5
import pandas as pd
data = pd.read_csv('data.csv')
data.to_hdf('data.h5', key='df', mode='w')
Saves a DataFrame to an HDF5 file.
41. Read HDF5 File
import pandas as pd
data = pd.read_hdf('data.h5', key='df')
print(data)
Reads data from an HDF5 file into a DataFrame.
42. Handle Missing Data with Interpolation
import pandas as pd
data = pd.read_csv('data.csv')
interpolated_data = data.interpolate()
print(interpolated_data)
Handles missing data by interpolation.
43. Calculate Correlation Matrix
import pandas as pd
data = pd.read_csv('data.csv')
correlation_matrix = data.corr()
print(correlation_matrix)
Calculates the correlation matrix of a DataFrame.
44. Create Random DataFrame
import pandas as pd
import numpy as np
data = pd.DataFrame(np.random.randn(5, 3), columns=['A', 'B', 'C'])
print(data)
Creates a DataFrame with random values.
45. Normalize Columns in DataFrame
import pandas as pd
from sklearn.preprocessing import MinMaxScaler
data = pd.read_csv('data.csv')
scaler = MinMaxScaler()
data[['Value']] = scaler.fit_transform(data[['Value']])
print(data)
Normalizes specific columns in a DataFrame.
46. Standardize Columns in DataFrame
import pandas as pd
from sklearn.preprocessing import StandardScaler
data = pd.read_csv('data.csv')
scaler = StandardScaler()
data[['Value']] = scaler.fit_transform(data[['Value']])
print(data)
Standardizes specific columns in a DataFrame.
47. Convert DataFrame to Dictionary
import pandas as pd
data = pd.read_csv('data.csv')
dict_data = data.to_dict()
print(dict_data)
Converts a DataFrame to a dictionary.
48. Load Data from a Web API
import requests
response = requests.get('https://api.example.com/data')
data = response.json()
print(data)
Loads data from a web API into a Python dictionary.
49. Save DataFrame to Google Sheets
import pandas as pd
import gspread
from oauth2client.service_account import ServiceAccountCredentials
scope = ["https://spreadsheets.google.com/feeds", "https://www.googleapis.com/auth/drive"]
creds = ServiceAccountCredentials.from_json_keyfile_name('credentials.json', scope)
client = gspread.authorize(creds)
sheet = client.open('SheetName').sheet1
data = pd.read_csv('data.csv')
sheet.update([data.columns.values.tolist()] + data.values.tolist())
Saves a DataFrame to a Google Sheet.
50. Read Data from Google Sheets
import gspread
from oauth2client.service_account import ServiceAccountCredentials
scope = ["https://spreadsheets.google.com/feeds", "https://www.googleapis.com/auth/drive"]
creds = ServiceAccountCredentials.from_json_keyfile_name('credentials.json', scope)
client = gspread.authorize(creds)
sheet = client.open('SheetName').sheet1
data = sheet.get_all_records()
print(data)
Reads data from a Google Sheet into a Python list.
51. Read SQL Data with SQLAlchemy
import pandas as pd
from sqlalchemy import create_engine
engine = create_engine('sqlite:///database.db')
data = pd.read_sql('SELECT * FROM table_name', engine)
print(data)
Reads data from a SQL database using SQLAlchemy.
52. Write Data to Google BigQuery
from google.cloud import bigquery
import pandas as pd
client = bigquery.Client()
data = pd.read_csv('data.csv')
client.load_table_from_dataframe(data, 'project.dataset.table')
Writes a DataFrame to Google BigQuery.
53. Load Data from Google BigQuery
from google.cloud import bigquery
client = bigquery.Client()
query = "SELECT * FROM `project.dataset.table`"
data = client.query(query).to_dataframe()
print(data)
Loads data from Google BigQuery into a DataFrame.
54. Convert DataFrame to HTML
import pandas as pd
data = pd.read_csv('data.csv')
html_data = data.to_html()
print(html_data)
Converts a DataFrame to an HTML table.
55. Read HTML Table into DataFrame
import pandas as pd
data = pd.read_html('table.html')[0]
print(data)
Reads an HTML table into a DataFrame.
56. Export DataFrame to LaTeX
import pandas as pd
data = pd.read_csv('data.csv')
latex_data = data.to_latex()
print(latex_data)
Exports a DataFrame to a LaTeX table format.
57. Create a DataFrame with Date Range
import pandas as pd
date_range = pd.date_range(start='2024-01-01', periods=5)
data = pd.DataFrame({'Date': date_range, 'Value': [10, 20, 30, 40, 50]})
print(data)
Creates a DataFrame with a date range.
58. Filter DataFrame by Date
import pandas as pd
data = pd.read_csv('data.csv', parse_dates=['Date'])
filtered_data = data[data['Date'] > '2024-01-01']
print(filtered_data)
Filters rows in a DataFrame based on a date condition.
59. Aggregate Data by Month
import pandas as pd
data = pd.read_csv('data.csv', parse_dates=['Date'])
monthly_data = data.resample('M', on='Date').sum()
print(monthly_data)
Aggregates data by month.
60. Create a DataFrame from a List of Dictionaries
import pandas as pd
data = [{'Name': 'John', 'Age': 28}, {'Name': 'Anna', 'Age': 24}]
df = pd.DataFrame(data)
print(df)
Creates a DataFrame from a list of dictionaries.
61. Flatten Nested Data in DataFrame
import pandas as pd
data = pd.read_csv('nested_data.csv')
flattened_data = pd.json_normalize(data['nested_column'])
print(flattened_data)
Flattens nested data in a DataFrame column.
62. Create DataFrame with Random Dates
import pandas as pd
import numpy as np
dates = pd.date_range(start='2024-01-01', periods=5)
data = pd.DataFrame({'Date': dates, 'Value': np.random.randn(5)})
print(data)
Creates a DataFrame with random dates and values.
63. Convert DataFrame to NumPy Array
import pandas as pd
data = pd.read_csv('data.csv')
array_data = data.to_numpy()
print(array_data)
Converts a DataFrame to a NumPy array.
64. Load Data from a CSV with Custom Delimiter
import pandas as pd
data = pd.read_csv('data.csv', delimiter=';')
print(data)
Reads a CSV file with a custom delimiter.
65. Handle Outliers in Data
import pandas as pd
data = pd.read_csv('data.csv')
filtered_data = data[data['Value'] < data['Value'].quantile(0.95)]
print(filtered_data)
Removes outliers by filtering extreme values.
66. Merge DataFrames with Outer Join
import pandas as pd
df1 = pd.DataFrame({'ID': [1, 2], 'Value': [10, 20]})
df2 = pd.DataFrame({'ID': [2, 3], 'Value': [30, 40]})
merged_df = pd.merge(df1, df2, on='ID', how='outer')
print(merged_df)
Merges DataFrames with an outer join.
67. Pivot and Aggregate Data
pythonCopier le codeimport pandas as pd
data = pd.read_csv('data.csv')
pivot_data = data.pivot_table(index='Category', values='Value', aggfunc='sum')
print(pivot_data)
Creates a pivot table and aggregates data.
68. Read Data from a Compressed File
import pandas as pd
data = pd.read_csv('data.csv.gz', compression='gzip')
print(data)
Reads data from a compressed CSV file.
69. Save DataFrame to Compressed File
import pandas as pd
data = pd.read_csv('data.csv')
data.to_csv('data_compressed.csv.gz', compression='gzip')
Saves a DataFrame to a compressed CSV file.
70. Create DataFrame with MultiIndex
import pandas as pd
arrays = [['A', 'A', 'B', 'B'], [1, 2, 1, 2]]
index = pd.MultiIndex.from_arrays(arrays, names=('Letter', 'Number'))
data = pd.DataFrame({'Value': [10, 20, 30, 40]}, index=index)
print(data)
Creates a DataFrame with a MultiIndex.
71. Calculate Rolling Statistics
import pandas as pd
data = pd.read_csv('data.csv', parse_dates=['Date'])
data.set_index('Date', inplace=True)
rolling_mean = data['Value'].rolling(window=3).mean()
print(rolling_mean)
Calculates rolling statistics for a DataFrame column.
72. Visualize Data with Matplotlib
import pandas as pd
import matplotlib.pyplot as plt
data = pd.read_csv('data.csv')
data.plot(x='Date', y='Value', kind='line')
plt.show()
Visualizes data with a line plot.
73. Handle Categorical Data
import pandas as pd
data = pd.read_csv('data.csv')
data['Category'] = data['Category'].astype('category')
print(data['Category'].cat.codes)
Converts categorical data to numerical codes.
74. Merge DataFrames with Different Column Names
import pandas as pd
df1 = pd.DataFrame({'ID': [1, 2], 'Value1': [10, 20]})
df2 = pd.DataFrame({'ID': [1, 2], 'Value2': [30, 40]})
merged_df = pd.merge(df1, df2, left_on='ID', right_on='ID')
print(merged_df)
Merges DataFrames with different column names.
75. Apply Function to DataFrame
import pandas as pd
data = pd.read_csv('data.csv')
data['Value'] = data['Value'].apply(lambda x: x * 2)
print(data)
Applies a function to a DataFrame column.
76. Create DataFrame with Date Range and Frequency
import pandas as pd
date_range = pd.date_range(start='2024-01-01', periods=10, freq='D')
data = pd.DataFrame({'Date': date_range, 'Value': range(10)})
print(data)
Creates a DataFrame with a date range and specified frequency.
77. Load Data from YAML
import pandas as pd
import yaml
with open('data.yaml', 'r') as file:
data = yaml.safe_load(file)
df = pd.DataFrame(data)
print(df)
Loads data from a YAML file into a DataFrame.
78. Save DataFrame to YAML
import pandas as pd
import yaml
data = pd.read_csv('data.csv')
data_dict = data.to_dict(orient='records')
with open('data.yaml', 'w') as file:
yaml.dump(data_dict, file)
Saves a DataFrame to a YAML file.
79. Handle Missing Data with Backward Fill
import pandas as pd
data = pd.read_csv('data.csv')
filled_data = data.fillna(method='bfill')
print(filled_data)
Handles missing data by backward fill.
80. Handle Missing Data with Mean Imputation
import pandas as pd
from sklearn.impute import SimpleImputer
data = pd.read_csv('data.csv')
imputer = SimpleImputer(strategy='mean')
data[['Value']] = imputer.fit_transform(data[['Value']])
print(data)
Imputes missing data with the mean of the column.
81. Create DataFrame with Hierarchical Index
import pandas as pd
arrays = [['A', 'A', 'B', 'B'], [1, 2, 1, 2]]
index = pd.MultiIndex.from_arrays(arrays, names=('Letter', 'Number'))
data = pd.DataFrame({'Value': [10, 20, 30, 40]}, index=index)
print(data)
Creates a DataFrame with a hierarchical index.
82. Filter Data by Multiple Conditions
import pandas as pd
data = pd.read_csv('data.csv')
filtered_data = data[(data['Age'] > 30) & (data['Value'] < 50)]
print(filtered_data)
Filters DataFrame rows based on multiple conditions.
83. Calculate Percentage Change
import pandas as pd
data = pd.read_csv('data.csv', parse_dates=['Date'])
data.set_index('Date', inplace=True)
data['Percentage Change'] = data['Value'].pct_change()
print(data)
Calculates the percentage change of a DataFrame column.
84. Create DataFrame with Random Integers
import pandas as pd
import numpy as np
data = pd.DataFrame({'Value': np.random.randint(0, 100, size=10)})
print(data)
Creates a DataFrame with random integers.
85. Create DataFrame with Sequential Dates
import pandas as pd
dates = pd.date_range(start='2024-01-01', periods=10)
data = pd.DataFrame({'Date': dates, 'Value': range(10)})
print(data)
Creates a DataFrame with sequential dates.
86. Save DataFrame to Feather Format
import pandas as pd
data = pd.read_csv('data.csv')
data.to_feather('data.feather')
Saves a DataFrame to a Feather file.
87. Read Feather File
import pandas as pd
data = pd.read_feather('data.feather')
print(data)
Reads data from a Feather file into a DataFrame.
88. Export DataFrame to CSV with Custom Header
import pandas as pd
data = pd.read_csv('data.csv')
data.to_csv('output.csv', header=['Column1', 'Column2'], index=False)
Exports a DataFrame to CSV with a custom header.
89. Create DataFrame from SQL Query
import pandas as pd
import sqlite3
conn = sqlite3.connect('database.db')
query = "SELECT Name, Age FROM users WHERE Age > 25"
data = pd.read_sql(query, conn)
print(data)
conn.close()
Creates a DataFrame from an SQL query.
90. Convert DataFrame to SQLite Table
import pandas as pd
import sqlite3
data = pd.read_csv('data.csv')
conn = sqlite3.connect('database.db')
data.to_sql('table_name', conn, if_exists='replace', index=False)
conn.close()
Converts a DataFrame to an SQLite table.
91. Remove Columns with All NaN Values
import pandas as pd
data = pd.read_csv('data.csv')
cleaned_data = data.dropna(axis=1, how='all')
print(cleaned_data)
Removes columns that contain only NaN values.
92. Create DataFrame with Random Normal Data
import pandas as pd
import numpy as np
data = pd.DataFrame({'Value': np.random.randn(10)})
print(data)
Creates a DataFrame with random normally distributed data.
93. Read Data from a Zip File
import pandas as pd
import zipfile
with zipfile.ZipFile('data.zip', 'r') as zip_ref:
zip_ref.extractall('data_folder')
data = pd.read_csv('data_folder/data.csv')
print(data)
Reads data from a file inside a zip archive.
94. Save DataFrame to Zip File
import pandas as pd
import zipfile
data = pd.read_csv('data.csv')
with zipfile.ZipFile('data.zip', 'w') as zip_ref:
data.to_csv('data_folder/data.csv', index=False)
zip_ref.write('data_folder/data.csv')
Saves a DataFrame to a file inside a zip archive.
95. Convert DataFrame to Python List
import pandas as pd
data = pd.read_csv('data.csv')
data_list = data.values.tolist()
print(data_list)
Converts a DataFrame to a Python list.
96. Perform DataFrame Operations in Parallel
import pandas as pd
from joblib import Parallel, delayed
data = pd.read_csv('data.csv')
def process_row(row):
return row * 2
results = Parallel(n_jobs=-1)(delayed(process_row)(row) for row in data['Value'])
print(results)
Performs operations on a DataFrame in parallel.
97. Create DataFrame from JSON File
import pandas as pd
data = pd.read_json('data.json')
print(data)
Creates a DataFrame from a JSON file.
98. Save DataFrame to JSON File
import pandas as pd
data = pd.read_csv('data.csv')
data.to_json('data.json')
Saves a DataFrame to a JSON file.
99. Load Data from Parquet File
import pandas as pd
data = pd.read_parquet('data.parquet')
print(data)
Loads data from a Parquet file into a DataFrame.
100. Save DataFrame to Parquet File
import pandas as pd
data = pd.read_csv('data.csv')
data.to_parquet('data.parquet')
Saves a DataFrame to a Parquet file.
This collection of 100 Python scripts for beginners is designed to enhance your understanding of cryptography with practical examples.
Post Comment