Top Python Projects for Beginners

Top Python Projects for Beginners
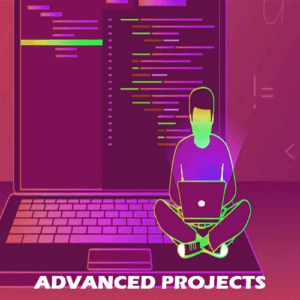
Password Cracker
Purpose: Develop a Python script capable of performing dictionary attacks and brute-force attacks on password-protected files, primarily targeting ZIP files. This project is intended for educational purposes to understand password cracking techniques.
Task: Write a Python program that:
- Cracks ZIP file passwords using brute-force attack and dictionary attack techniques.
- Implements a brute-force attack by trying all possible combinations of characters up to a specified length.
- Performs a dictionary attack using a list of commonly used passwords.
- Provides insights into password security and the importance of strong passwords.

Advanced Phishing Website
Purpose: Develop a sophisticated phishing website to capture login credentials.
Task: Write a Python program that:
- Creates a phishing website mimicking a popular login page.
- Uses Flask to serve the phishing website.
- Logs the captured credentials to a file for later retrieval.

Full-Stack Web Application with User Authentication, Database, and API Integration
Purpose: Build a complex full-stack web application using Python, Flask, SQLAlchemy, and third-party APIs. This project includes user authentication, database operations, and integration with an external API to fetch and display data.
Task: Write a Python program that:
- Creates a Flask web application with user registration and login functionality.
- Connects to a SQLite database to store user information and their preferences.
- Integrates with a third-party API (e.g., OpenWeatherMap) to fetch and display weather information based on user preferences.
- Implements a user interface using HTML, CSS, and JavaScript.

Network Packet Sniffer
Purpose: Build a network packet sniffer to capture, decode, and display various types of network packets. This project involves working with raw sockets, understanding network protocols, and implementing packet parsing.
Task: Write a Python program that:
- Captures network packets from a specified network interface.
- Decodes and analyzes the packet headers for Ethernet, IP, TCP, UDP, and ICMP protocols.
- Displays detailed information about each captured packet.

Python Keylogger
Purpose: Develop a Python script that acts as a keylogger, capturing keystrokes from the user’s keyboard. This project is for educational purposes only and should not be used for any malicious intent.
Task: Write a Python program that:
- Captures keystrokes from the user’s keyboard.
- Records the keys pressed, including special keys like Enter, Backspace, Shift, etc.
- Logs the captured keystrokes to a text file.

Python Botnet
Purpose: Develop a Python script to create a basic botnet for educational purposes only. A botnet is a network of compromised computers, each of which is called a bot. The bots are controlled by a central server, called the command and control (C&C) server. Again, remember, this is for educational purposes only and should not be used for any malicious activities.
Task: Write a Python program that:
- Creates a server to act as the C&C server.
- Sets up a botnet network with multiple bots.
- Sends commands from the C&C server to the bots and executes them on the bots’ machines.
- Receives output from the bots and displays it on the C&C server.

Network Vulnerability Scanner
Purpose: Develop a Python script for scanning network hosts and identifying potential vulnerabilities.
Task: Write a Python program that:
- Discovers hosts in a given IP range.
- Scans discovered hosts for open ports.
- Conducts banner grabbing to identify services running on open ports.
- Performs vulnerability assessment based on the identified services.
- Generates a report outlining potential vulnerabilities.

DNS Spoofing Tool
Purpose: Develop a Python script to perform DNS spoofing attacks.
Task: Write a Python program that:
- Intercepts DNS requests and responds with fake DNS replies.
- Redirects traffic from legitimate servers to fake servers controlled by the attacker.
- Captures sensitive information exchanged between the victim and the fake server.
ARP Spoofing Tool
Purpose: Develop a Python script to perform ARP spoofing attacks.
Task: Write a Python program that:
- Sends ARP packets to the target and router, spoofing the MAC addresses.
- Captures packets from the target and router, forwarding them to the appropriate recipient.
- Allows the attacker to intercept and modify network traffic.

Advanced Keylogger
Purpose: Develop an advanced keylogger in Python that captures keystrokes and logs them to a file. Additionally, it will take periodic screenshots and send the log file and screenshots to an email address.
Task: Write a Python program that:
- Captures keystrokes and logs them.
- Takes periodic screenshots.
- Sends the log file and screenshots to a specified email address at regular intervals.
Post Comment