Plane Game In Python

Introduction
Creating a plane game offers an exciting way to engage players with aerial combat or flight simulation experiences. In this type of game, players typically control a plane, navigating through various challenges, avoiding obstacles, and sometimes engaging in combat or missions. The gameplay often involves maneuvering the plane through different environments, such as skies filled with enemy aircraft, weather hazards, or intricate terrains.
The core mechanics of a plane game generally include controlling the plane’s movement, managing its speed and altitude, and using weapons or tools to achieve objectives. The game may feature diverse levels or missions, each with unique challenges and goals. Graphics and sound effects are crucial in creating an immersive experience, enhancing the sensation of flying and engaging players in dynamic aerial action. Whether aiming for a realistic simulation or an arcade-style adventure, a well-designed plane game combines skillful controls, strategic gameplay, and captivating visuals to deliver an enjoyable and thrilling experience.
Coding a Breakout Game in Python
Coding a plane game in Python involves several key steps to bring the game to life. The process starts with setting up the game environment using a library like Pygame, which provides tools for handling graphics, input, and sound.
First, you create the plane and enemy sprites, along with their movement mechanics. The plane, controlled by the player, is typically moved with keyboard inputs, while enemies might have predefined movement patterns or follow the plane. Next, you implement collision detection to manage interactions between the plane and enemies, ensuring that game events like scoring or game-over conditions are triggered appropriately.
The game loop, which is the core of the game, continuously updates the game state, processes player inputs, and redraws the screen. Additionally, incorporating features such as scoring, different levels, and sound effects can enhance the gaming experience. By organizing the code into classes and functions, you make the game easier to manage and extend, allowing for a more engaging and polished final product.

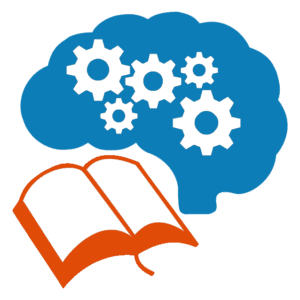
What You Need To Know
To successfully code a plane game in Python, there are several essential elements you need to understand:
Programming Fundamentals: A solid grasp of Python programming basics, including variables, control structures, functions, and object-oriented programming, is crucial. This foundational knowledge will help you structure your game efficiently.
Game Development Libraries: Familiarity with game development libraries like Pygame is necessary. Pygame provides tools for handling graphics, sound, and user input, which are vital for creating an interactive game.
Graphics Handling: Understanding how to manage and render game assets, such as images and animations, is important. You’ll need to know how to load, display, and move these assets within the game window.
Event Handling: Learning how to handle user inputs, such as keyboard and mouse events, will allow you to control the plane and interact with other game elements.
Collision Detection: Implementing collision detection is essential for managing interactions between the plane and obstacles or enemies. This involves calculating when two objects intersect and responding accordingly.
Game Loop Structure: Knowing how to set up and manage the game loop is key. The game loop continuously updates the game state, processes input, and refreshes the screen to create a smooth gaming experience.
Physics and Movement: Basic understanding of physics concepts such as velocity, acceleration, and gravity can help you create more realistic movements for the plane and other game elements.
Scoring and Progression: Implementing a scoring system and managing game progression, including levels and difficulty, can enhance the gameplay experience and provide goals for the player.
Debugging and Testing: Skills in debugging and testing will help you identify and fix issues in your code, ensuring the game runs smoothly and meets your expectations.


Plane Game With Python (Full Code)
import pygame
import sys
import random
# Initialize Pygame
pygame.init()
# Set up the screen
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("Plane Game")
# Load images
plane_img = pygame.image.load("plane.png").convert_alpha()
enemy_img = pygame.image.load("enemy.png").convert_alpha()
bullet_img = pygame.image.load("bullet.png").convert_alpha()
enemy_bullet_img = pygame.image.load("enemy_bullet.png").convert_alpha()
# Load background image
background_img = pygame.image.load("background.png").convert()
# Background attributes
background_img = pygame.transform.scale(background_img, (SCREEN_WIDTH, SCREEN_HEIGHT))
bg_x = 0
# Background scroll speed
bg_speed = 2
# Background color
BACKGROUND_COLOR = (135, 206, 235) # Sky blue
# Plane attributes
plane_rect = plane_img.get_rect(midleft=(50, SCREEN_HEIGHT // 2))
plane_speed = 5
# Bullet attributes
bullet_speed = 8
bullets = []
# Enemy attributes
enemy_speed = 3
enemies = []
enemy_timer = pygame.time.get_ticks()
# Enemy bullet attributes
enemy_bullet_speed = 6
enemy_bullets = []
# Game over flag
game_over = False
# Score
score = 0
# Main game loop
clock = pygame.time.Clock()
while True:
# Event handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
bullet_rect = bullet_img.get_rect(midleft=(plane_rect.right, plane_rect.centery))
bullets.append(bullet_rect)
# Move plane
keys = pygame.key.get_pressed()
if keys[pygame.K_UP]:
plane_rect.y -= plane_speed
if keys[pygame.K_DOWN]:
plane_rect.y += plane_speed
# Check for boundaries
if plane_rect.top <= 0:
plane_rect.top = 0
if plane_rect.bottom >= SCREEN_HEIGHT:
plane_rect.bottom = SCREEN_HEIGHT
# Spawn enemies
if pygame.time.get_ticks() - enemy_timer > 1500:
enemy_rect = enemy_img.get_rect(midright=(random.randint(SCREEN_WIDTH + 20, SCREEN_WIDTH + 100), random.randint(50, SCREEN_HEIGHT - 50)))
enemies.append(enemy_rect)
enemy_timer = pygame.time.get_ticks()
# Move enemies
for enemy in enemies:
enemy.x -= enemy_speed
if enemy.right < 0:
enemies.remove(enemy)
# Move bullets
for bullet in bullets:
bullet.x += bullet_speed
if bullet.left > SCREEN_WIDTH:
bullets.remove(bullet)
# Move enemy bullets
for enemy_bullet in enemy_bullets:
enemy_bullet.x -= enemy_bullet_speed
if enemy_bullet.right < 0:
enemy_bullets.remove(enemy_bullet)
# Enemies fire bullets
for enemy in enemies:
if random.randint(1, 100) == 1:
enemy_bullet_rect = enemy_bullet_img.get_rect(midright=(enemy.left, enemy.centery))
enemy_bullets.append(enemy_bullet_rect)
# Check for collision with enemies
for enemy in enemies:
if plane_rect.colliderect(enemy):
game_over = True
for bullet in bullets:
if bullet.colliderect(enemy):
bullets.remove(bullet)
enemies.remove(enemy)
score += 10
# Check for collision with enemy bullets
for enemy_bullet in enemy_bullets:
if plane_rect.colliderect(enemy_bullet):
game_over = True
# Scroll background
bg_x -= bg_speed
if bg_x <= -SCREEN_WIDTH:
bg_x = 0
# Draw background
screen.blit(background_img, (bg_x, 0))
screen.blit(background_img, (bg_x + SCREEN_WIDTH, 0))
# Draw plane
screen.blit(plane_img, plane_rect)
# Draw bullets
for bullet in bullets:
screen.blit(bullet_img, bullet)
# Draw enemy bullets
for enemy_bullet in enemy_bullets:
screen.blit(enemy_bullet_img, enemy_bullet)
# Draw enemies
for enemy in enemies:
screen.blit(enemy_img, enemy)
# Draw score and information
font = pygame.font.Font(None, 24)
score_text = font.render("Score: " + str(score), True, (255, 255, 255))
screen.blit(score_text, (10, 10))
info_text = font.render("Press SPACE to fire", True, (255, 255, 255))
screen.blit(info_text, (10, 40))
# Update the display
pygame.display.flip()
# Set the frame rate
clock.tick(60)
# Check for game over
if game_over:
# Game over text
font = pygame.font.Font(None, 64)
game_over_text = font.render("Game Over!", True, (255, 0, 0))
game_over_rect = game_over_text.get_rect(center=(SCREEN_WIDTH // 2, SCREEN_HEIGHT // 2))
screen.blit(game_over_text, game_over_rect)
pygame.display.flip()
pygame.time.wait(2000) # Wait 2 seconds before exiting
pygame.quit()
sys.exit()

Main Part Of The Code To Understand
Importing Libraries and Initializing Games:
import pygame
import sys
import random
# Initialize Pygame
pygame.init()
# Set up the screen
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("Plane Game")
Here, we define the dimensions of the game window (800 pixels wide and 600 pixels high) and create a screen surface using pygame.display.set_mode(). We also set the window caption to “Plane Game” using pygame.display.set_caption().

Loading Images:
# Load images
plane_img = pygame.image.load("plane.png").convert_alpha()
enemy_img = pygame.image.load("enemy.png").convert_alpha()
bullet_img = pygame.image.load("bullet.png").convert_alpha()
enemy_bullet_img = pygame.image.load("enemy_bullet.png").convert_alpha()
These lines load the images for the plane, enemies, bullets, and enemy bullets respectively. The convert_alpha() function optimizes the images for faster rendering.

Bullets Attributes:
# Bullet attributes
bullet_speed = 8
bullets = []
bullet_speed defines the speed of bullets, and bullets is a list that will store the positions of all bullets fired by the plane.

Enemy Attributes:
# Enemy attributes
enemy_speed = 3
enemies = []
enemy_timer = pygame.time.get_ticks()
enemy_speed represents the speed at which enemies move across the screen. enemies is a list to hold the positions of all enemies, and enemy_timer keeps track of when to spawn new enemies.

Enemy Bullet Attributes:
# Enemy bullet attributes
enemy_bullet_speed = 6
enemy_bullets = []
enemy_bullet_speed sets the speed of bullets fired by enemies, and enemy_bullets stores the positions of those bullets.

Game Over Flag:
# Game over flag
game_over = False
# Score
score = 0
game_over is a boolean flag that indicates whether the game is over, and score keeps track of the player’s score.

Main Loop:
while True:
# Event handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
bullet_rect = bullet_img.get_rect(midleft=(plane_rect.right, plane_rect.centery))
bullets.append(bullet_rect)
- This part of the code checks for events such as key presses, mouse clicks, etc.
- The
for
loop iterates over all the events that have occurred since the last frame. - If the event is a
QUIT
event (like clicking the close button of the window), the game exits gracefully usingpygame.quit()
andsys.exit()
. - If a key is pressed (the
KEYDOWN
event), it checks if the key is the spacebar (pygame.K_SPACE
). If so, it creates a new bullet at the position of the plane and adds it to thebullets
list.

Moving The Plane:
# Move plane
keys = pygame.key.get_pressed()
if keys[pygame.K_UP]:
plane_rect.y -= plane_speed
if keys[pygame.K_DOWN]:
plane_rect.y += plane_speed
- This part moves the plane up and down based on the player’s input (up arrow key and down arrow key).
- It checks for which keys are currently being pressed using
pygame.key.get_pressed()
. - If the up arrow key is pressed, it moves the plane up (
plane_rect.y -= plane_speed
). - If the down arrow key is pressed, it moves the plane down (
plane_rect.y += plane_speed
). - It also checks if the plane is at the top or bottom of the screen and prevents it from going out of bounds.

Spawning Enemies:
# Spawn enemies
if pygame.time.get_ticks() - enemy_timer > 1500:
enemy_rect = enemy_img.get_rect(midright=(random.randint(SCREEN_WIDTH + 20, SCREEN_WIDTH + 100), random.randint(50, SCREEN_HEIGHT - 50)))
enemies.append(enemy_rect)
enemy_timer = pygame.time.get_ticks()
- This section spawns enemies at regular intervals (every 1500 milliseconds or 1.5 seconds).
- It creates a new enemy rectangle and positions it randomly on the right side of the screen, at a random height.

Moving Enemies:
# Move enemies
for enemy in enemies:
enemy.x -= enemy_speed
if enemy.right < 0:
enemies.remove(enemy)
- This loop moves each enemy towards the left side of the screen.
- If an enemy moves completely off the left side of the screen, it is removed from the
enemies
list.

Moving Bullets:
# Move bullets
for bullet in bullets:
bullet.x += bullet_speed
if bullet.left > SCREEN_WIDTH:
bullets.remove(bullet)
- This loop moves each bullet fired by the plane towards the right side of the screen.
- If a bullet moves completely off the right side of the screen, it is removed from the
bullets
list.

Moving Enemy Bullets:
# Move enemy bullets
for enemy_bullet in enemy_bullets:
enemy_bullet.x -= enemy_bullet_speed
if enemy_bullet.right < 0:
enemy_bullets.remove(enemy_bullet)
- This loop moves each bullet fired by the enemies towards the left side of the screen.
- If an enemy bullet moves completely off the left side of the screen, it is removed from the
enemy_bullets
list.

Enemies Firing Bullets:
# Enemies fire bullets
for enemy in enemies:
if random.randint(1, 100) == 1:
enemy_bullet_rect = enemy_bullet_img.get_rect(midright=(enemy.left, enemy.centery))
enemy_bullets.append(enemy_bullet_rect)
- This loop iterates over all enemies.
- For each enemy, there’s a 1% chance (
random.randint(1, 100) == 1
) that it will fire a bullet. - If an enemy decides to fire, a new bullet rectangle is created at the position of the enemy’s left side and its center vertically, and it’s added to the
enemy_bullets
list.

Collision Detection:
# Check for collision with enemies
for enemy in enemies:
if plane_rect.colliderect(enemy):
game_over = True
for bullet in bullets:
if bullet.colliderect(enemy):
bullets.remove(bullet)
enemies.remove(enemy)
score += 10
# Check for collision with enemy bullets
for enemy_bullet in enemy_bullets:
if plane_rect.colliderect(enemy_bullet):
game_over = True
- These loops check for collisions between:
- The plane and enemies. If the plane collides with any enemy, it sets
game_over
toTrue
. - Bullets fired by the plane and enemies. If a bullet fired by the player collides with an enemy, it removes the bullet and the enemy from their respective lists and increases the score by 10.
- The plane and enemy bullets. If the plane collides with any enemy bullet, it sets
game_over
toTrue
.
- The plane and enemies. If the plane collides with any enemy, it sets

Scrolling Background:
# Scroll background
bg_x -= bg_speed
if bg_x <= -SCREEN_WIDTH:
bg_x = 0
- This part scrolls the background to create a sense of movement.
bg_x
keeps track of the background’s x-coordinate. It is decremented bybg_speed
, causing the background to move to the left.- When the background moves completely off the screen to the left, it’s reset to its original position to create an illusion of continuous scrolling.
- The background is drawn twice to cover the entire width of the screen.

Drawing Elements:
# Draw background
screen.blit(background_img, (bg_x, 0))
screen.blit(background_img, (bg_x + SCREEN_WIDTH, 0))
# Draw plane
screen.blit(plane_img, plane_rect)
# Draw bullets
for bullet in bullets:
screen.blit(bullet_img, bullet)
# Draw enemy bullets
for enemy_bullet in enemy_bullets:
screen.blit(enemy_bullet_img, enemy_bullet)
# Draw enemies
for enemy in enemies:
screen.blit(enemy_img, enemy)
# Draw score and information
font = pygame.font.Font(None, 24)
score_text = font.render("Score: " + str(score), True, (255, 255, 255))
screen.blit(score_text, (10, 10))
info_text = font.render("Press SPACE to fire", True, (255, 255, 255))
screen.blit(info_text, (10, 40))
- This section draws the plane, bullets, enemy bullets, enemies, score, and information on the screen.

Updating Display and Frame Rate:
# Update the display
pygame.display.flip()
# Set the frame rate
clock.tick(60)
# Check for game over
if game_over:
# Game over text
font = pygame.font.Font(None, 64)
game_over_text = font.render("Game Over!", True, (255, 0, 0))
game_over_rect = game_over_text.get_rect(center=(SCREEN_WIDTH // 2, SCREEN_HEIGHT // 2))
screen.blit(game_over_text, game_over_rect)
pygame.display.flip()
pygame.time.wait(2000) # Wait 2 seconds before exiting
pygame.quit()
sys.exit()
This code handles displaying a “Game Over” message, waiting for 2 seconds, and then quitting the game.

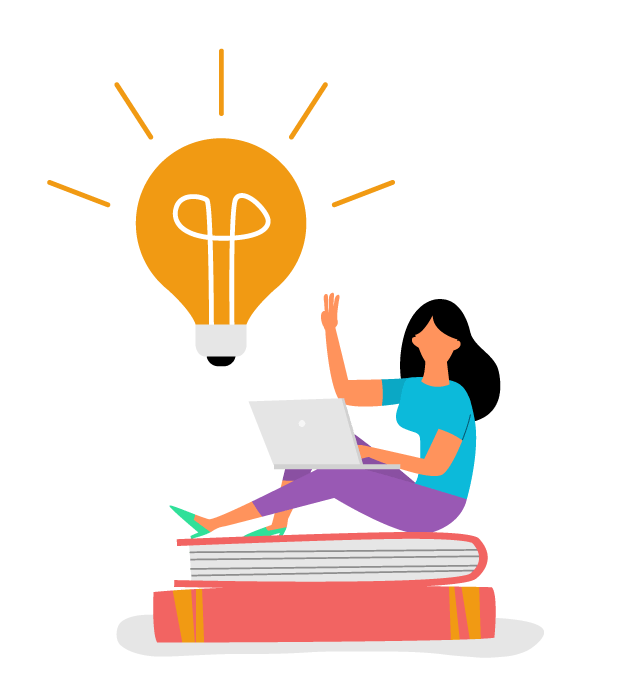
Summary
The code for the plane game integrates key elements to create an engaging and interactive experience. By setting up the game window, defining the dimensions, and initializing essential components, we established the foundation for gameplay. The game involves handling player input, managing game objects, and updating their positions, all while ensuring smooth graphics rendering and collision detection.
The use of Pygame’s functionalities facilitates real-time game dynamics, allowing players to control the plane and interact with the game environment. Overall, this structured approach provides a solid basis for developing a functional and entertaining plane game.
2 comments